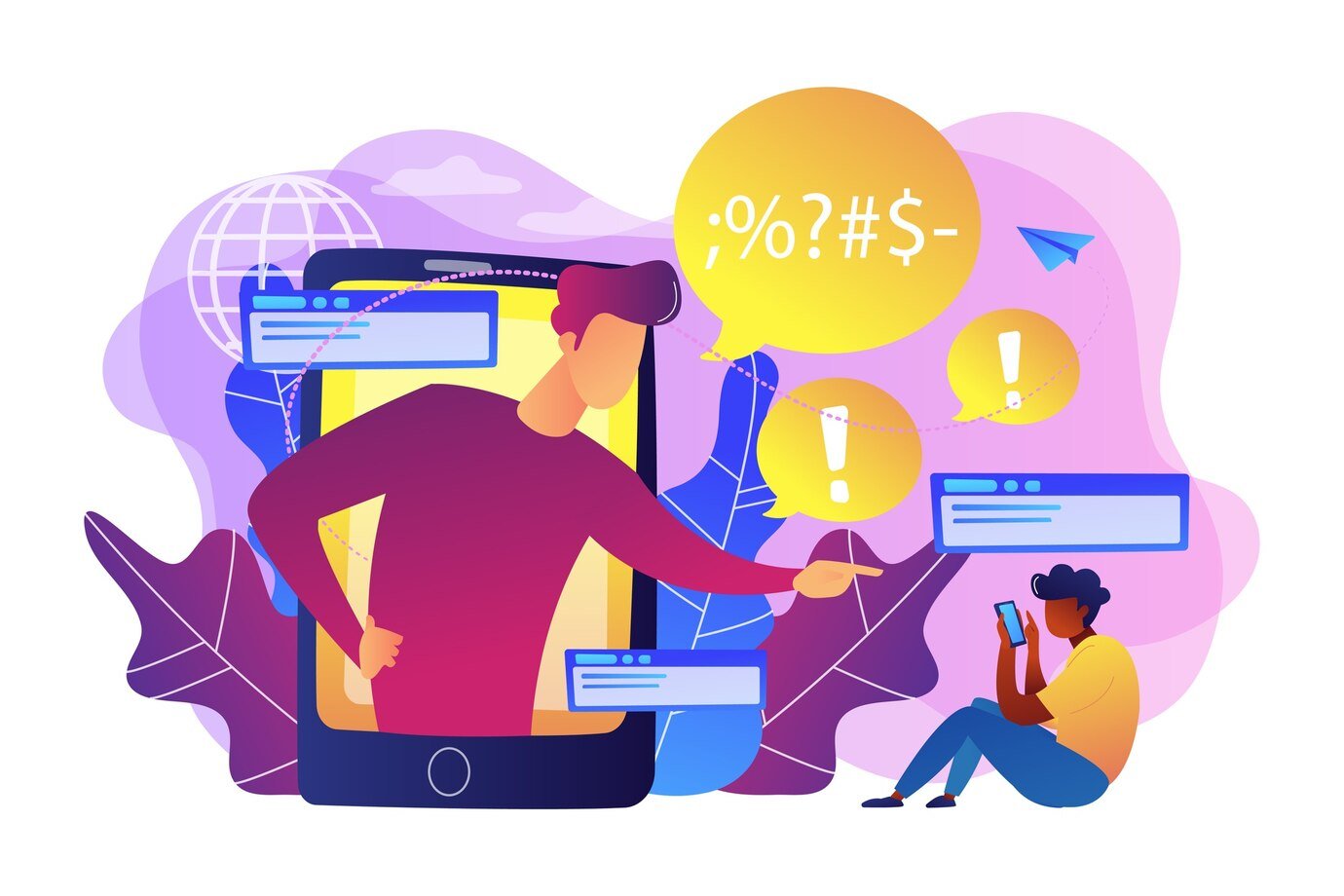
Common Flutter Development Mistakes and How to Avoid Them
Common Flutter Development Mistakes and How to Avoid Them
Thanks to its fast development process, beautiful UI components, and strong community support, Flutter has become one of the most popular frameworks for building cross-platform applications. However, like any development framework, Flutter comes with its own set of challenges. In this blog, we’ll explore some of the most common Flutter development mistakes and how you can avoid them.
1. Not Understanding the Flutter Widget Tree Mistake:
Many beginners fail to understand the widget tree structure, leading to inefficient app performance. Every UI element in Flutter is a widget, and improper structuring can lead to unnecessary rebuilds, affecting app performance.
Solution:
-
Use const constructors for widgets that don’t change frequently.
-
Leverage the const keyword to prevent unwanted widget rebuilds.
-
Use StatefulWidget only when necessary and prefer StatelessWidget when possible.
2. Poor State Management Mistake:
Managing the app state incorrectly can lead to complex, unmanageable code and performance issues.
Solution:
-
Choose a state management approach suitable for your project (Provider, Riverpod, Bloc, Redux, etc.).
-
Keep UI and business logic separate by structuring your code properly.
-
Avoid excessive use of setState(), especially in large widgets.
3. Blocking the Main Thread Mistake:
Performing heavy operations (like database queries or API calls) on the main UI thread can cause the app to lag.
Solution:
-
Use Future and async/await to handle asynchronous operations properly.
-
Utilize Isolates for CPU-intensive tasks to prevent UI freezing.
-
Use compute() for running heavy functions in a separate thread.
4. Ignoring Code Optimization Mistake:
Writing unoptimized code can lead to slow performance and increased memory consumption.
Solution:
-
Optimize build() methods by breaking them into smaller widgets.
-
Avoid unnecessary widget nesting.
-
Use ListView.builder() instead of ListView when working with large lists to improve performance.
5. Improper Handling of Null Safety Mistake:
Since Dart is null-safe, failing to handle null values correctly can lead to unexpected crashes.
Solution:
-
Use late, ? and ! operators wisely.
-
Initialize variables properly to prevent null errors.
-
Handle potential null values using if checks or ?? (null-coalescing operator).
6. Not Using Hot Reload Efficiently Mistake:
Many developers don’t take full advantage of Flutter’s hot reload, leading to slower development cycles.
Solution:
-
Always use hot reload instead of restarting the app completely to speed up testing.
-
If changes don’t reflect, use hot restart when necessary.
-
Use logs and debugging tools to track errors efficiently.
7. Ignoring Platform-Specific Code Mistake:
Flutter is cross-platform, but some features still require platform-specific implementation, which is often overlooked.
Solution:
-
Use Platform Channels to communicate with native code when necessary.
-
Check platform-specific dependencies before implementation.
-
Test your app on both iOS and Android devices for compatibility.
8. Not Testing the Application Properly Mistake:
Skipping testing or relying only on manual testing can lead to undetected bugs.
Solution:
-
Write unit tests to check business logic.
-
Implement widget tests to verify UI behavior.
-
Use integration tests to ensure the app functions correctly as a whole.
9. Hardcoding Strings and Colors Mistake:
Hardcoding text and colors makes it difficult to scale, localize, or theme the app.
Solution:
-
Store strings in a separate localization file to support multiple languages.
-
Use ThemeData to manage app-wide colors and styles.
-
Follow Flutter’s Material Theme to maintain consistency.
10. Not Keeping Up with Flutter Updates Mistake:
Flutter is constantly evolving, and failing to update your dependencies can lead to compatibility issues.
Solution:
-
Regularly check for updates in pub.dev.
-
Upgrade dependencies using Flutter pub upgrade.
-
Follow Flutter’s official documentation and changelogs to stay updated.
If you want to study flutter courses in short period, Contact us
Conclusion
Avoiding these common mistakes will help you build efficient, high-performance Flutter applications with cleaner code. Whether you’re a beginner or an experienced developer, following best practices will make your development process smoother and more productive. Keep learning, stay updated, and happy coding!